Networking using NSURLSession
Mon 05 Oct 2015, 07:12
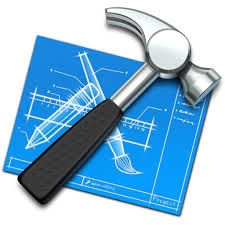
This is my code for downloading image or json (you can modify if you need another format) using NSURLSession, this code based on sample code from https://developer.apple.com/library/ios/samplecode/LazyTableImages/Introduction/Intro.html:
DownloaderRecord.h
#import <Foundation/Foundation.h>
#import <UIKit/UIKit.h>
@interface DownloaderRecord : NSObject
@property (nonatomic, strong) NSString *url;
@property (nonatomic, strong) NSString *postData;
@property (nonatomic, strong) UIImage *imageData;
@property (nonatomic, strong) NSDictionary *jsonData;
@end
DownloaderRecord.m
#import "DownloaderRecord.h"
@implementation DownloaderRecord
@end
Downloader.h
#import <Foundation/Foundation.h>
@class DownloaderRecord;
@interface Downloader : NSObject
@property (nonatomic, strong) DownloaderRecord *downloaderRecord;
@property (nonatomic, copy) void (^completionHandler)(void);
- (void)startDownloadImage;
- (void)startDownloadJson;
- (void)cancelDownload;
@end
Downloader.m
#import "Downloader.h"
#import "DownloaderRecord.h"
#define kAppIconSize 48
@interface Downloader ()
@property (nonatomic, strong) NSURLSessionDataTask *sessionTask;
@end
@implementation Downloader
// -------------------------------------------------------------------------------
// startDownloadImage
// -------------------------------------------------------------------------------
- (void)startDownloadImage
{
NSURLRequest *request = [NSURLRequest requestWithURL:[NSURL URLWithString:self.downloaderRecord.url]];
// create an session data task to obtain and download the app icon
_sessionTask = [[NSURLSession sharedSession] dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
// in case we want to know the response status code
//NSInteger HTTPStatusCode = [(NSHTTPURLResponse *)response statusCode];
if (error != nil)
{
if ([error code] == NSURLErrorAppTransportSecurityRequiresSecureConnection)
{
// if you get error NSURLErrorAppTransportSecurityRequiresSecureConnection (-1022),
// then your Info.plist has not been properly configured to match the target server.
//
abort();
}
}
[[NSOperationQueue mainQueue] addOperationWithBlock: ^{
// Set appIcon and clear temporary data/image
UIImage *image = [[UIImage alloc] initWithData:data];
if (image.size.width != kAppIconSize || image.size.height != kAppIconSize)
{
CGSize itemSize = CGSizeMake(kAppIconSize, kAppIconSize);
UIGraphicsBeginImageContextWithOptions(itemSize, NO, 0.0f);
CGRect imageRect = CGRectMake(0.0, 0.0, itemSize.width, itemSize.height);
[image drawInRect:imageRect];
self.downloaderRecord.imageData = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
}
else
{
self.downloaderRecord.imageData = image;
}
// call our completion handler to tell our client that our icon is ready for display
if (self.completionHandler != nil)
{
self.completionHandler();
}
}];
}];
[self.sessionTask resume];
}
// -------------------------------------------------------------------------------
// startDownloadJson
// -------------------------------------------------------------------------------
- (void)startDownloadJson
{
NSURL *url=[NSURL URLWithString:self.downloaderRecord.url];
NSData *postData = [self.downloaderRecord.postData dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%lu",(unsigned long)[postData length]];
NSMutableURLRequest *request = [[NSMutableURLRequest alloc] init];
[request setURL:url];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-length"];
[request setHTTPBody:postData];
_sessionTask =
[[NSURLSession sharedSession]
dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error != nil)
{
if ([error code] == NSURLErrorAppTransportSecurityRequiresSecureConnection)
{
abort();
}
}else{
[[NSOperationQueue mainQueue] addOperationWithBlock: ^{
self.downloaderRecord.jsonData=[NSJSONSerialization JSONObjectWithData:data options:NSJSONReadingMutableContainers error:nil];
if (self.completionHandler != nil)
{
self.completionHandler();
}
}];
}
}];
[self.sessionTask resume];
}
// -------------------------------------------------------------------------------
// cancelDownload
// -------------------------------------------------------------------------------
- (void)cancelDownload
{
[self.sessionTask cancel];
_sessionTask = nil;
}
@end
This is how to use the code in UITableViewCell:
ViewController.h
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource,UITableViewDelegate>
@property (weak, nonatomic) IBOutlet UITableView *tblView;
@property (copy,nonatomic) NSDictionary *tblData;
@end
ViewController.m
#import "ViewController.h"
#import "DownloaderRecord.h"
#import "Downloader.h"
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
DownloaderRecord *downloaderRecord=[[DownloaderRecord alloc] init];
downloaderRecord.url=@"http://www.yourhost.com/yourJsonAPI.Anything";
downloaderRecord.postData=[[NSString alloc] initWithFormat:@"postVariable=%@”,];
Downloader *downloader = [[Downloader alloc] init];
downloader.downloaderRecord = downloaderRecord;
[downloader setCompletionHandler:^{
self.tblData = downloaderRecord.jsonData;
[self.tblView reloadData];
}];
[downloader startDownloadJson];
}
@end
You can download the code from http://www.ariyanki.net/download/#
0
10243 views